Hello,
We can do this using custom JS code. Sample code along with explanation is given below:
Code:
const checkInterval = 500;
const maxAttempts = 10;
let attempts = 0;
while (attempts < maxAttempts) {
const el = document.querySelectorAll(elementIdentifier);
if (el[1] !== undefined ) {
return el[1];
}
await new Promise((resolve) => setTimeout(resolve, checkInterval));
attempts++;
}
return null;
};
const tableMainFunction = async() =>{
var tableEl = await tableElementChecker("#hi-report-505fcc06 table");
console.log(tableEl);
tableEl.addEventListener("click", function(e) {
var cellValue = e.target.textContent.trim();
console.log("Clicked value: " + cellValue);
var generatedUrl = "https://www.google.com/search?q=" + encodeURIComponent(cellValue);
window.open(generatedUrl, "_blank");
});
}
tableMainFunction();
Usage:
-
Create a table report with required columns.
-
Hover on 3 dots beside Filters
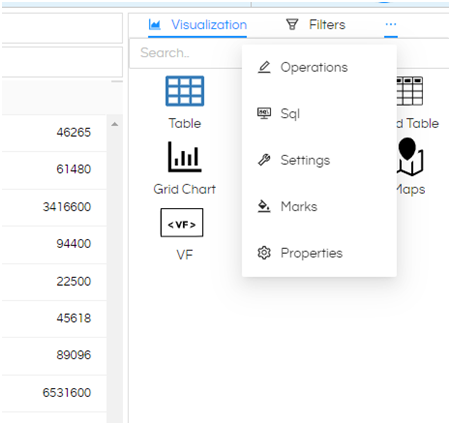
-
Select Operations from the dropdown
-
Select JS
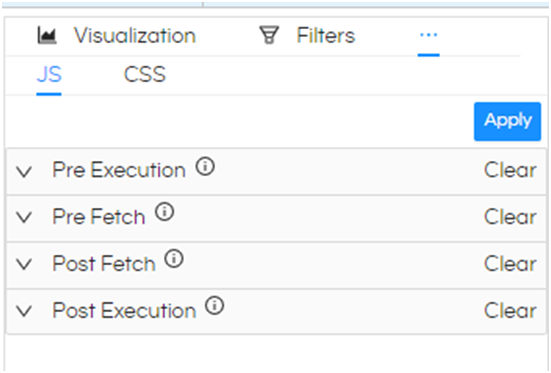
- In JS Expand Post Fetch and paste the code in it
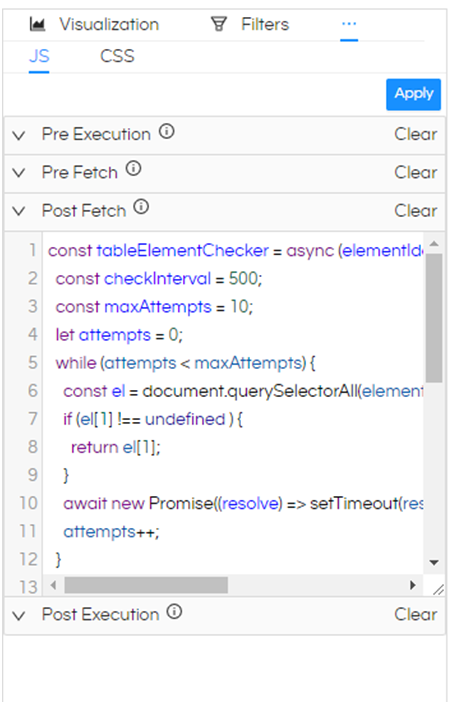
- After pasting the code click on Apply and generate the report.
- Now if you click on any cell in the table then that value will be searched in the google.
- Here you can modify the code in a way that rather than searching in google. You can append the value to any URL and trigger that URL.
Code Explanation:
var tableEl = await tableElementChecker("#hi-report-505fcc06 table");
Here *“#hi-report-505fcc06"*is the id of the report and it will be different for every report. Please update the same accordingly.
You can find this id in Operations, select CSS. There you will find the id.
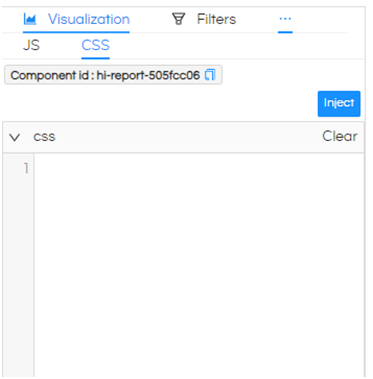
Component id is the id we need, Once you get this you can update the code with this id.
var generatedUrl = “Google” + encodeURIComponent(cellValue);
Here instead “Google” , you can have your own URL string to that you can add cell value.
Example: “URL string” + cellValue
window.open(generatedUrl, “_blank”);
This line will open the generated URL in new tab.
Thank you,
Helical Insight.